DCTag SDK
1.2.1
Copyright © 1983-2016 Advantech Co., Ltd.
|
DCTag.h
Go to the documentation of this file.
104 int (*read_tag) (
112 int (*write_tag) (
120 int (*update_tag) (
int dc_tag_remove(DC_TAG_HANDLE tag_handle)
Remove a tag from the DataCenter which previous added by yourself.
Definition: DCTag.c:1507
DC_TAG_HANDLE dc_tag_add(char const *tag_name, void *tag_context)
Add a tag to the DataCenter.
Definition: DCTag.c:1493
Definition: DCTag.h:54
void * dc_tag_add_subscription(DC_TAG_HANDLE tag_handles[], uint32_t tag_count)
Subscribe tags for read.
Definition: DCTag.c:1562
char const * dc_tag_explain_quality(int16_t quality)
obtain explanations for the specified quality value.
Definition: DCTag.c:1994
int dc_tag_read(DC_TAG_HANDLE tag_handles[], DC_TAG tag_values[], uint32_t tag_count)
Read one or more tags.
Definition: DCTag.c:1716
DC_TAG_HANDLE dc_tag_open(char const *tag_name, void *tag_context)
Open a exist tag in the DataCenter with the specified name.
Definition: DCTag.c:1519
int dc_tag_write(DC_TAG_HANDLE tag_handles[], double tag_values[], uint32_t tag_count)
Write one or more tags.
Definition: DCTag.c:1783
Definition: DCTag.h:62
Definition: DCTag.h:48
int dc_tag_close(DC_TAG_HANDLE tag_handle)
Close a tag from the DataCenter which previous opened by yourself.
Definition: DCTag.c:1551
int dc_tag_remove_subscription(void *subscription)
Remove a previous subscription.
Definition: DCTag.c:1603
Definition: DCTag.h:98
Definition: DCTag.h:56
Definition: DCTag.h:58
int dc_tag_update(DC_TAG_HANDLE tag_handles[], DC_TAG tag_values[], uint32_t tag_count)
Update one or more tags.
Definition: DCTag.c:1893
Definition: DCTag.h:60
Definition: DCTag.h:52
Definition: DCTag.h:50
int dc_tag_read_subscription(void *subscription, DC_TAG tag_values[], uint32_t tag_count)
Read one or more tags by previous subscription.
Definition: DCTag.c:1649
int dc_tag_init(uint32_t max_tag_count, DC_TAG_CALLBACK *callback, void *user_context)
Initialize the DCTag SDK.
Definition: DCTag.c:1329
Definition: DCTag.h:64
Definition: DCTag.h:80
Definition: DCTag.h:46
Generated on Thu May 19 2016 14:18:17 for DCTag SDK by
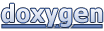