![]() |
OpenNI 1.5.4
|
XnStackT.h
Go to the documentation of this file.
00001 #ifndef _XN_STACK_T_H_ 00002 #define _XN_STACK_T_H_ 00003 00004 //--------------------------------------------------------------------------- 00005 // Includes 00006 //--------------------------------------------------------------------------- 00007 #include "XnListT.h" 00008 00009 //--------------------------------------------------------------------------- 00010 // Code 00011 //--------------------------------------------------------------------------- 00012 template<class T, class TAlloc = XnLinkedNodeDefaultAllocatorT<T> > 00013 class XnStackT : protected XnListT<T, TAlloc> 00014 { 00015 public: 00016 typedef XnListT<T, TAlloc> Base; 00017 00018 typedef typename Base::ConstIterator ConstIterator; 00019 00020 XnStackT() : Base() {} 00021 00022 XnStackT(const XnStackT& other) : Base() 00023 { 00024 *this = other; 00025 } 00026 00027 XnStackT& operator=(const XnStackT& other) 00028 { 00029 Base::operator=(other); 00030 // no other members 00031 return *this; 00032 } 00033 00034 ~XnStackT() {} 00035 00036 XnBool IsEmpty() const { return Base::IsEmpty(); } 00037 00038 XnStatus Push(T const& value) { return Base::AddFirst(value); } 00039 00040 XnStatus Pop(T& value) 00041 { 00042 ConstIterator it = Begin(); 00043 if (it == End()) 00044 { 00045 return XN_STATUS_IS_EMPTY; 00046 } 00047 value = *it; 00048 return Base::Remove(it); 00049 } 00050 00051 T const& Top() const { return *Begin(); } 00052 T& Top() { return *Begin(); } 00053 00054 ConstIterator Begin() const { return Base::Begin(); } 00055 ConstIterator End() const { return Base::End(); } 00056 }; 00057 00058 #endif // _XN_STACK_T_H_
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
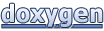