![]() |
OpenNI 1.5.4
|
XnModuleCppRegistratration.h
Go to the documentation of this file.
00001 /**************************************************************************** 00002 * * 00003 * OpenNI 1.x Alpha * 00004 * Copyright (C) 2011 PrimeSense Ltd. * 00005 * * 00006 * This file is part of OpenNI. * 00007 * * 00008 * OpenNI is free software: you can redistribute it and/or modify * 00009 * it under the terms of the GNU Lesser General Public License as published * 00010 * by the Free Software Foundation, either version 3 of the License, or * 00011 * (at your option) any later version. * 00012 * * 00013 * OpenNI is distributed in the hope that it will be useful, * 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of * 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * 00016 * GNU Lesser General Public License for more details. * 00017 * * 00018 * You should have received a copy of the GNU Lesser General Public License * 00019 * along with OpenNI. If not, see <http://www.gnu.org/licenses/>. * 00020 * * 00021 ****************************************************************************/ 00022 #ifndef __XN_MODULE_CPP_REGISTRATION_H__ 00023 #define __XN_MODULE_CPP_REGISTRATION_H__ 00024 00025 //--------------------------------------------------------------------------- 00026 // Includes 00027 //--------------------------------------------------------------------------- 00028 #include "XnModuleCppInterface.h" 00029 #include <XnUtils.h> 00030 00031 using namespace xn; 00032 00033 //--------------------------------------------------------------------------- 00034 // Internal Macros 00035 //--------------------------------------------------------------------------- 00037 #define _XN_MODULE_INST g_pTheModule 00038 00039 #define _CONCAT(a,b) a##b 00040 00041 inline XnModuleNodeHandle __ModuleNodeToHandle(xn::ModuleProductionNode* pNode) 00042 { 00043 return ((XnModuleNodeHandle)pNode); 00044 } 00045 00046 #define __XN_EXPORT_NODE_COMMON(ExportedClass, ExportedName, Type) \ 00047 \ 00048 static ExportedClass* ExportedName = new ExportedClass(); \ 00049 \ 00050 void XN_CALLBACK_TYPE _CONCAT(ExportedClass,GetDescription)(XnProductionNodeDescription* pDescription) \ 00051 { \ 00052 ExportedName->GetDescription(pDescription); \ 00053 } \ 00054 \ 00055 XnStatus XN_CALLBACK_TYPE _CONCAT(ExportedClass,EnumerateProductionTrees) \ 00056 (XnContext* pContext, XnNodeInfoList* pTreesList, XnEnumerationErrors* pErrors) \ 00057 { \ 00058 Context context(pContext); \ 00059 NodeInfoList list(pTreesList); \ 00060 EnumerationErrors errors(pErrors); \ 00061 return ExportedName->EnumerateProductionTrees(context, list, pErrors == NULL ? NULL : &errors); \ 00062 } \ 00063 \ 00064 XnStatus XN_CALLBACK_TYPE _CONCAT(ExportedClass,Create)(XnContext* pContext, \ 00065 const XnChar* strInstanceName, \ 00066 const XnChar* strCreationInfo, \ 00067 XnNodeInfoList* pNeededTrees, \ 00068 const XnChar* strConfigurationDir, \ 00069 XnModuleNodeHandle* phInstance) \ 00070 { \ 00071 xn::NodeInfoList* pNeeded = NULL; \ 00072 if (pNeededTrees != NULL) \ 00073 { \ 00074 pNeeded = XN_NEW(xn::NodeInfoList, pNeededTrees); \ 00075 } \ 00076 ModuleProductionNode* pNode; \ 00077 Context context(pContext); \ 00078 XnStatus nRetVal = ExportedName->Create(context, strInstanceName, strCreationInfo, \ 00079 pNeeded, strConfigurationDir, &pNode); \ 00080 if (nRetVal != XN_STATUS_OK) \ 00081 { \ 00082 XN_DELETE(pNeeded); \ 00083 return (nRetVal); \ 00084 } \ 00085 *phInstance = __ModuleNodeToHandle(pNode); \ 00086 XN_DELETE(pNeeded); \ 00087 return (XN_STATUS_OK); \ 00088 } \ 00089 \ 00090 void XN_CALLBACK_TYPE _CONCAT(ExportedClass,Destroy)(XnModuleNodeHandle hInstance) \ 00091 { \ 00092 ModuleProductionNode* pNode = (ModuleProductionNode*)hInstance; \ 00093 ExportedName->Destroy(pNode); \ 00094 } \ 00095 \ 00096 void XN_CALLBACK_TYPE _CONCAT(ExportedClass,GetExportedInterface)( \ 00097 XnModuleExportedProductionNodeInterface* pInterface) \ 00098 { \ 00099 pInterface->GetDescription = _CONCAT(ExportedClass,GetDescription); \ 00100 pInterface->EnumerateProductionTrees = _CONCAT(ExportedClass,EnumerateProductionTrees); \ 00101 pInterface->Create = _CONCAT(ExportedClass,Create); \ 00102 pInterface->Destroy = _CONCAT(ExportedClass,Destroy); \ 00103 pInterface->GetInterface.General = __ModuleGetGetInterfaceFunc(Type); \ 00104 } \ 00105 \ 00106 static XnStatus _CONCAT(ExportedClass,RegisterResult) = \ 00107 _XN_MODULE_INST->AddExportedNode(_CONCAT(ExportedClass,GetExportedInterface)); 00108 00109 #define _XN_EXPORT_NODE_COMMON(ExportedClass, Type) \ 00110 __XN_EXPORT_NODE_COMMON(ExportedClass, _g_##ExportedClass, Type) 00111 00112 //--------------------------------------------------------------------------- 00113 // Forward Declarations 00114 //--------------------------------------------------------------------------- 00115 00116 void XN_CALLBACK_TYPE __ModuleGetProductionNodeInterface(XnModuleProductionNodeInterface* pInterface); 00117 void XN_CALLBACK_TYPE __ModuleGetDeviceInterface(XnModuleDeviceInterface* pInterface); 00118 void XN_CALLBACK_TYPE __ModuleGetGeneratorInterface(XnModuleGeneratorInterface* pInterface); 00119 void XN_CALLBACK_TYPE __ModuleGetMapGeneratorInterface(XnModuleMapGeneratorInterface* pInterface); 00120 void XN_CALLBACK_TYPE __ModuleGetDepthGeneratorInterface(XnModuleDepthGeneratorInterface* pInterface); 00121 void XN_CALLBACK_TYPE __ModuleGetImageGeneratorInterface(XnModuleImageGeneratorInterface* pInterface); 00122 void XN_CALLBACK_TYPE __ModuleGetIRGeneratorInterface(XnModuleIRGeneratorInterface* pInterface); 00123 void XN_CALLBACK_TYPE __ModuleGetUserGeneratorInterface(XnModuleUserGeneratorInterface* pInterface); 00124 void XN_CALLBACK_TYPE __ModuleGetHandsGeneratorInterface(XnModuleHandsGeneratorInterface* pInterface); 00125 void XN_CALLBACK_TYPE __ModuleGetGestureGeneratorInterface(XnModuleGestureGeneratorInterface* pInterface); 00126 void XN_CALLBACK_TYPE __ModuleGetSceneAnalyzerInterface(XnModuleSceneAnalyzerInterface* pInterface); 00127 void XN_CALLBACK_TYPE __ModuleGetAudioGeneratorInterface(XnModuleAudioGeneratorInterface* pInterface); 00128 void XN_CALLBACK_TYPE __ModuleGetRecorderInterface(XnModuleRecorderInterface* pInterface); 00129 void XN_CALLBACK_TYPE __ModuleGetPlayerInterface(XnModulePlayerInterface* pInterface); 00130 void XN_CALLBACK_TYPE __ModuleGetCodecInterface(XnModuleCodecInterface* pInterface); 00131 void XN_CALLBACK_TYPE __ModuleGetScriptNodeInterface(XnModuleScriptNodeInterface* pInterface); 00132 00133 //--------------------------------------------------------------------------- 00134 // Utility Macros 00135 //--------------------------------------------------------------------------- 00136 00137 typedef void (XN_CALLBACK_TYPE *GetInterfaceFuncPtr)(void* pInterface); 00138 00139 static GetInterfaceFuncPtr __ModuleGetGetInterfaceFunc(XnProductionNodeType type) 00140 { 00141 // start with concrete type 00142 if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_DEVICE)) 00143 return (GetInterfaceFuncPtr)__ModuleGetDeviceInterface; 00144 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_DEPTH)) 00145 return (GetInterfaceFuncPtr)__ModuleGetDepthGeneratorInterface; 00146 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_IMAGE)) 00147 return (GetInterfaceFuncPtr)__ModuleGetImageGeneratorInterface; 00148 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_IR)) 00149 return (GetInterfaceFuncPtr)__ModuleGetIRGeneratorInterface; 00150 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_USER)) 00151 return (GetInterfaceFuncPtr)__ModuleGetUserGeneratorInterface; 00152 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_GESTURE)) 00153 return (GetInterfaceFuncPtr)__ModuleGetGestureGeneratorInterface; 00154 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_SCENE)) 00155 return (GetInterfaceFuncPtr)__ModuleGetSceneAnalyzerInterface; 00156 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_AUDIO)) 00157 return (GetInterfaceFuncPtr)__ModuleGetAudioGeneratorInterface; 00158 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_RECORDER)) 00159 return (GetInterfaceFuncPtr)__ModuleGetRecorderInterface; 00160 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_PLAYER)) 00161 return (GetInterfaceFuncPtr)__ModuleGetPlayerInterface; 00162 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_HANDS)) 00163 return (GetInterfaceFuncPtr)__ModuleGetHandsGeneratorInterface; 00164 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_CODEC)) 00165 return (GetInterfaceFuncPtr)__ModuleGetCodecInterface; 00166 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_SCRIPT)) 00167 return (GetInterfaceFuncPtr)__ModuleGetScriptNodeInterface; 00168 // and continue with abstract ones 00169 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_MAP_GENERATOR)) 00170 return (GetInterfaceFuncPtr)__ModuleGetMapGeneratorInterface; 00171 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_GENERATOR)) 00172 return (GetInterfaceFuncPtr)__ModuleGetGeneratorInterface; 00173 else if (xnIsTypeDerivedFrom(type, XN_NODE_TYPE_PRODUCTION_NODE)) 00174 return (GetInterfaceFuncPtr)__ModuleGetProductionNodeInterface; 00175 00176 // unknown 00177 XN_ASSERT(FALSE); 00178 return NULL; 00179 } 00180 00182 #if XN_PLATFORM_SUPPORTS_DYNAMIC_LIBS 00183 #define XN_EXPORT_MODULE(ModuleClass) \ 00184 \ 00185 ModuleClass __moduleInstance; \ 00186 Module* _XN_MODULE_INST = &__moduleInstance; 00187 #else 00188 #define XN_EXPORT_MODULE(ModuleClass) \ 00189 \ 00190 static ModuleClass __moduleInstance; \ 00191 static Module* _XN_MODULE_INST = &__moduleInstance; 00192 #endif 00193 00195 #define XN_EXPORT_NODE(ExportedClass, nodeType) \ 00196 _XN_EXPORT_NODE_COMMON(ExportedClass, nodeType) 00197 00199 #define XN_EXPORT_DEVICE(ExportedClass) \ 00200 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_DEVICE) 00201 00203 #define XN_EXPORT_DEPTH(ExportedClass) \ 00204 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_DEPTH) 00205 00207 #define XN_EXPORT_IMAGE(ExportedClass) \ 00208 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_IMAGE) 00209 00211 #define XN_EXPORT_IR(ExportedClass) \ 00212 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_IR) 00213 00214 #define XN_EXPORT_USER(ExportedClass) \ 00215 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_USER) 00216 00217 #define XN_EXPORT_HANDS(ExportedClass) \ 00218 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_HANDS) 00219 00220 #define XN_EXPORT_GESTURE(ExportedClass) \ 00221 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_GESTURE) 00222 00223 #define XN_EXPORT_SCENE(ExportedClass) \ 00224 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_SCENE) 00225 00227 #define XN_EXPORT_AUDIO(ExportedClass) \ 00228 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_AUDIO) 00229 00231 #define XN_EXPORT_RECORDER(ExportedClass) \ 00232 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_RECORDER) 00233 00235 #define XN_EXPORT_PLAYER(ExportedClass) \ 00236 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_PLAYER) 00237 00238 #define XN_EXPORT_CODEC(ExportedClass) \ 00239 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_CODEC) 00240 00241 #define XN_EXPORT_SCRIPT(ExportedClass) \ 00242 _XN_EXPORT_NODE_COMMON(ExportedClass, XN_NODE_TYPE_SCRIPT) 00243 00244 //--------------------------------------------------------------------------- 00245 // Exported C functions 00246 //--------------------------------------------------------------------------- 00247 #if XN_PLATFORM_SUPPORTS_DYNAMIC_LIBS 00248 #include <XnModuleCFunctions.h> 00249 #define XN_MODULE_FUNC_TYPE XN_C_API_EXPORT 00250 extern Module* _XN_MODULE_INST; 00251 #else 00252 #define XN_MODULE_FUNC_TYPE static 00253 static Module* _XN_MODULE_INST; 00254 #endif 00255 00256 XN_MODULE_FUNC_TYPE XnStatus XN_C_DECL XN_MODULE_LOAD() 00257 { 00258 XnStatus nRetVal = XN_STATUS_OK; 00259 00260 nRetVal = _XN_MODULE_INST->Load(); 00261 XN_IS_STATUS_OK(nRetVal); 00262 00263 return (XN_STATUS_OK); 00264 } 00265 00266 XN_MODULE_FUNC_TYPE void XN_C_DECL XN_MODULE_UNLOAD() 00267 { 00268 _XN_MODULE_INST->Unload(); 00269 } 00270 00271 XN_MODULE_FUNC_TYPE XnUInt32 XN_C_DECL XN_MODULE_GET_EXPORTED_NODES_COUNT() 00272 { 00273 return _XN_MODULE_INST->GetExportedNodesCount(); 00274 } 00275 00276 XN_MODULE_FUNC_TYPE XnStatus XN_C_DECL XN_MODULE_GET_EXPORTED_NODES_ENTRY_POINTS(XnModuleGetExportedInterfacePtr* aEntryPoints, XnUInt32 nCount) 00277 { 00278 return _XN_MODULE_INST->GetExportedNodes(aEntryPoints, nCount); 00279 } 00280 00281 XN_MODULE_FUNC_TYPE void XN_C_DECL XN_MODULE_GET_OPEN_NI_VERSION(XnVersion* pVersion) 00282 { 00283 pVersion->nMajor = XN_MAJOR_VERSION; 00284 pVersion->nMinor = XN_MINOR_VERSION; 00285 pVersion->nMaintenance = XN_MAINTENANCE_VERSION; 00286 pVersion->nBuild = XN_BUILD_VERSION; 00287 } 00288 00289 #if !XN_PLATFORM_SUPPORTS_DYNAMIC_LIBS 00290 #include <XnUtils.h> 00291 00292 static XnOpenNIModuleInterface moduleInterface = 00293 { 00294 XN_MODULE_LOAD, 00295 XN_MODULE_UNLOAD, 00296 XN_MODULE_GET_EXPORTED_NODES_COUNT, 00297 XN_MODULE_GET_EXPORTED_NODES_ENTRY_POINTS, 00298 XN_MODULE_GET_OPEN_NI_VERSION 00299 }; 00300 static XnStatus registerResult = xnRegisterModuleWithOpenNI(&moduleInterface, NULL, __FILE__); 00301 #endif 00302 00303 #endif // __XN_MODULE_CPP_REGISTRATION_H__
Generated on Wed May 16 2012 10:16:05 for OpenNI 1.5.4 by
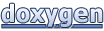